Lab06 Instructions
User Manual:
Open the PDF directly: View PDF .
Page Count: 4
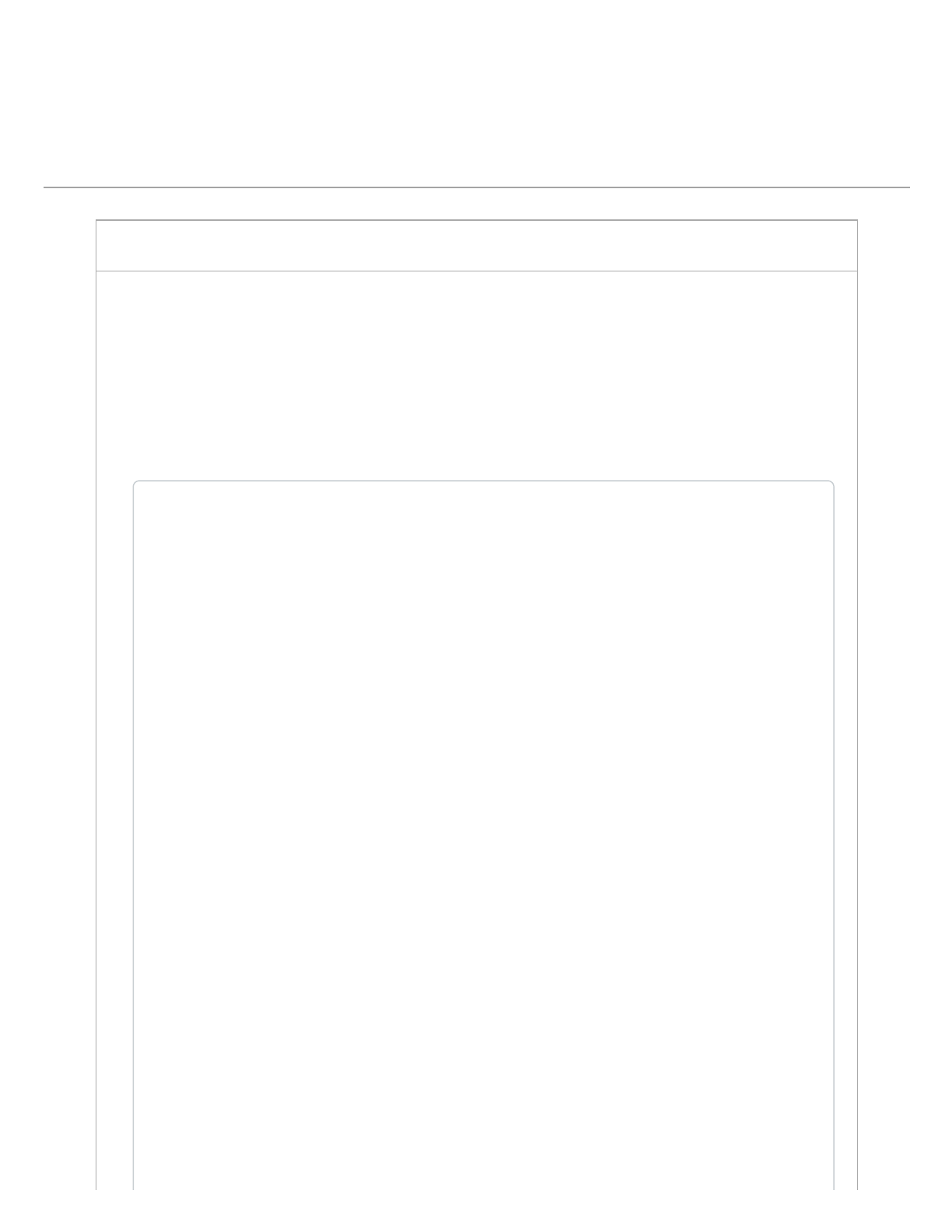
2/26/2019 Quiz: Lab 06
https://cilearn.csuci.edu/courses/6978/quizzes/15478/take/questions/239479 1/4
Lab06
Started:Feb26at12:35pm
QuizInstrucons
100ptsQuestion1
TaskDescripon
ImplementasolutiontotheDiningPhilosophersproblemusingthepthreadlibrary's
mutexandconditionalvariablefunctions.
Recallthepseudocodefromthelecturenotes:
monitor DiningPhilisophers {
enum { THINKING; HUNGRY; EATING} state[5];
condition self[5];
void pickup (int i)
{
state[i] = HUNGRY;
test(i);
if (state[i] != EATING)
self[i].wait();
}
void putdown (int i)
{
state[i] = THINKING;
// test left and right neighbors
test((i + 4) % 5);
test((i + 1) % 5);
}
void test (int i)
{
if ( (state[(i + 4) % 5] != EATING) &&
(state[i] == HUNGRY) && (state[(i + 1) % 5] != EATING) )
{
state[i] = EATING ;
self[i].signal();
}
}
initialization_code()
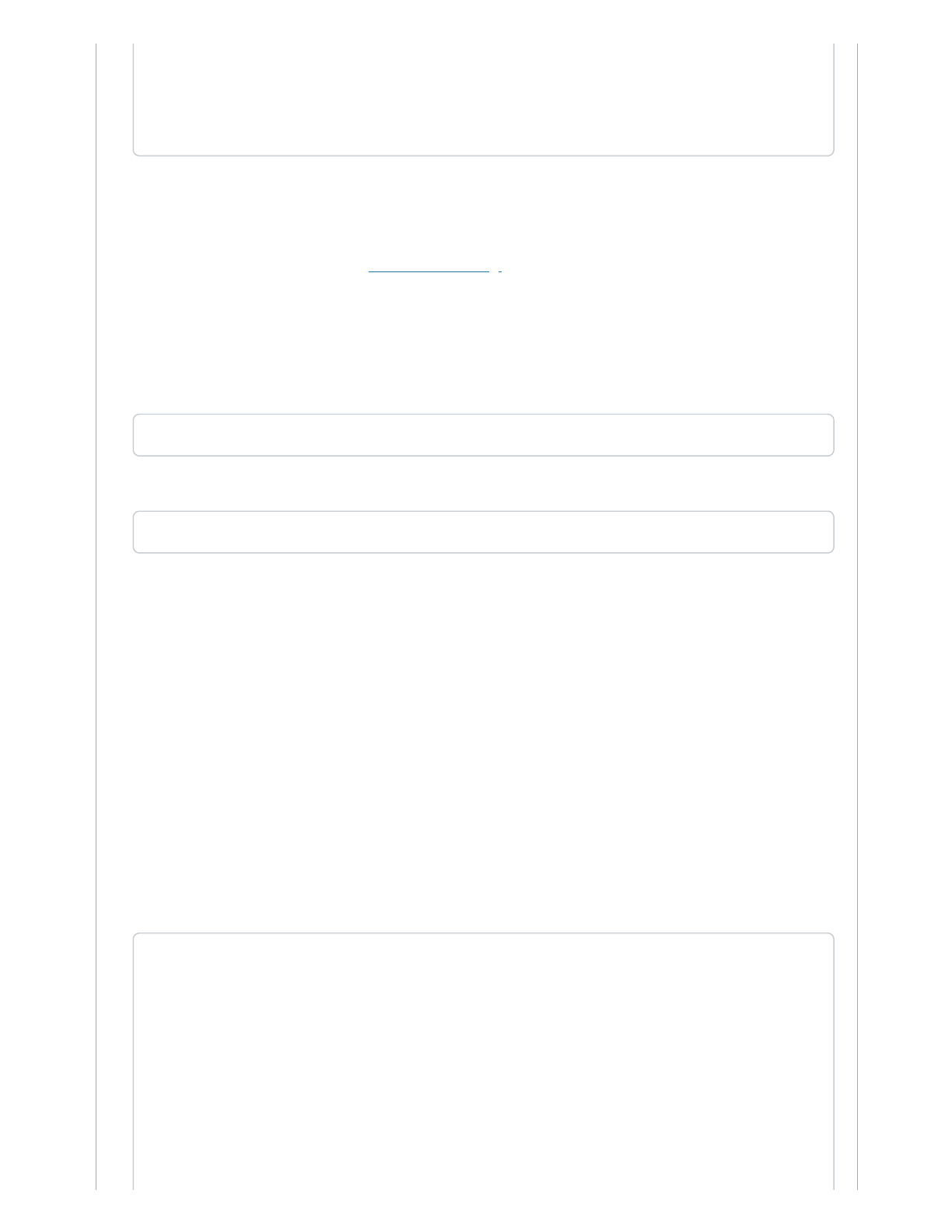
2/26/2019 Quiz: Lab 06
https://cilearn.csuci.edu/courses/6978/quizzes/15478/take/questions/239479 2/4
{
for (int i = 0; i < 5; i++)
state[i] = THINKING;
}
}
Implementaon
ThecontentofthearchivefilephiloBase.zipisanaiveimplementationofthedining
philosophersparadigm.Analyzethecode.Doyouseeanyproblemswiththecode?
Compileandrunthecodeanumberoftimes.Atsomepointtheprogramwillhang.
Ifitdoesnot,thenexperimentwithsomelargervaluesforthenumberofseatsandthe
numberofrounds.Addthefollowinglinebetweenthelockingrequestsforchopsticks:
usleep(DELAY*2);
Addasimilarlinebetweenthecallstoreturnthechopsticks:
usleep(DELAY*4);
Experimentwiththelengthofthedelay.
Canyouexplainwhytheprogramgetsstuck?
Onewaytofixtheproblemistoutilizepthread_mutex_trylock()withspinning.That
impliesusingtheapproachinwhichpickingbothchopsticksisattempted,butifonlyone
isavailable,thentheothermustbereturned;nodeadlockswilloccuroccurs.Tryitout.
Abetterapproachistousemonitors.Cdoesnotprovidemonitors,soyouwillimplement
them.Amonitorsynchronizesexecutionofitsmemberfunctions,soonlyonefunction
canbeexecutingatanygiventime.Thesameeffectcanbeachievedwithpthread
mutexes.ThefollowingisapseudocodethataddsaCfunctiontoavirtualmonitor
definedbyamutex:
function()
{
wait(monitor_mutex);
...
// body of the function
...
signal(monitor_mutex);
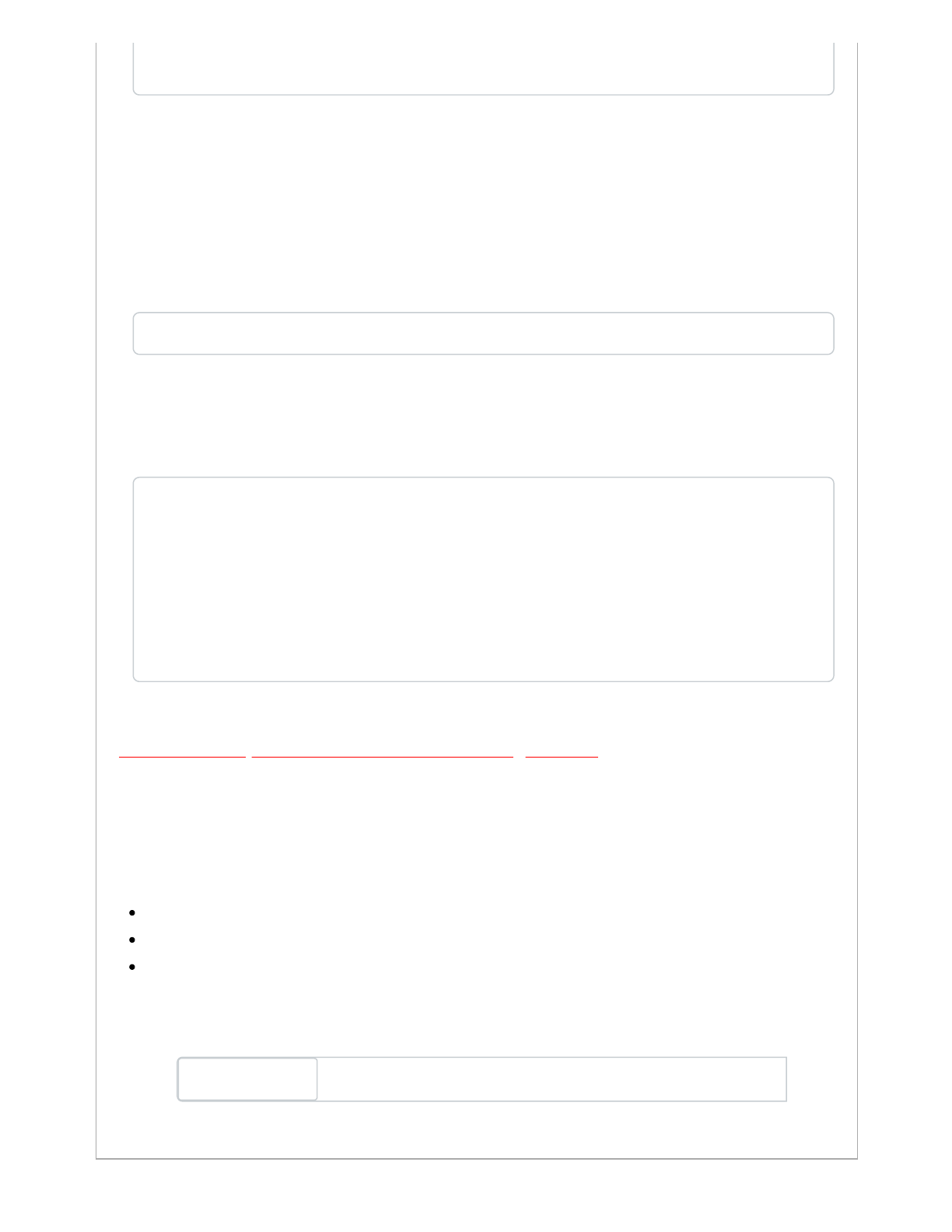
2/26/2019 Quiz: Lab 06
https://cilearn.csuci.edu/courses/6978/quizzes/15478/take/questions/239479 3/4
Upload
}
Youwillneedjustonemonitorforthisassignment,sojustonemutexissufficientforall
functions.
Notethataconditionalvariablemustbeassociatedwithamutex.Itwillbeanerrortocall
waitorsignalonaconditionalvariablefromoutsideofacriticalsectionprotectedbythe
associatedmutex.
Also,youcanallocateamutexstaticallyinthefollowingway:
pthread_mutex_t monitor_mutex = PTHREAD_MUTEX_INITIALIZER;
Notefurthermore,thattryingtolockthesamelocktwiceblocksthethread.
Withthepickup()andputdown()functionssynchronizedwithamonitor,each
philosopherisathreadusingthefollowingtemplate:
...
pickup(i);
// EAT
putdown(i);
...
Generatearandomnumberanduseitinadelayinlieuoftheeatingtime.
NOTE:YourimplementationMUSTfollowthisguideline.
Submission
Youmustsubmitthefollowing:
thesignedsourcecode,
theMakeLists.txtfilewithwhichyoubuiltyourapplication,and
multiplescriptsofteststhatconfirmthatyourprogramdoesnotlockunderany
condition.
ChooseaFile
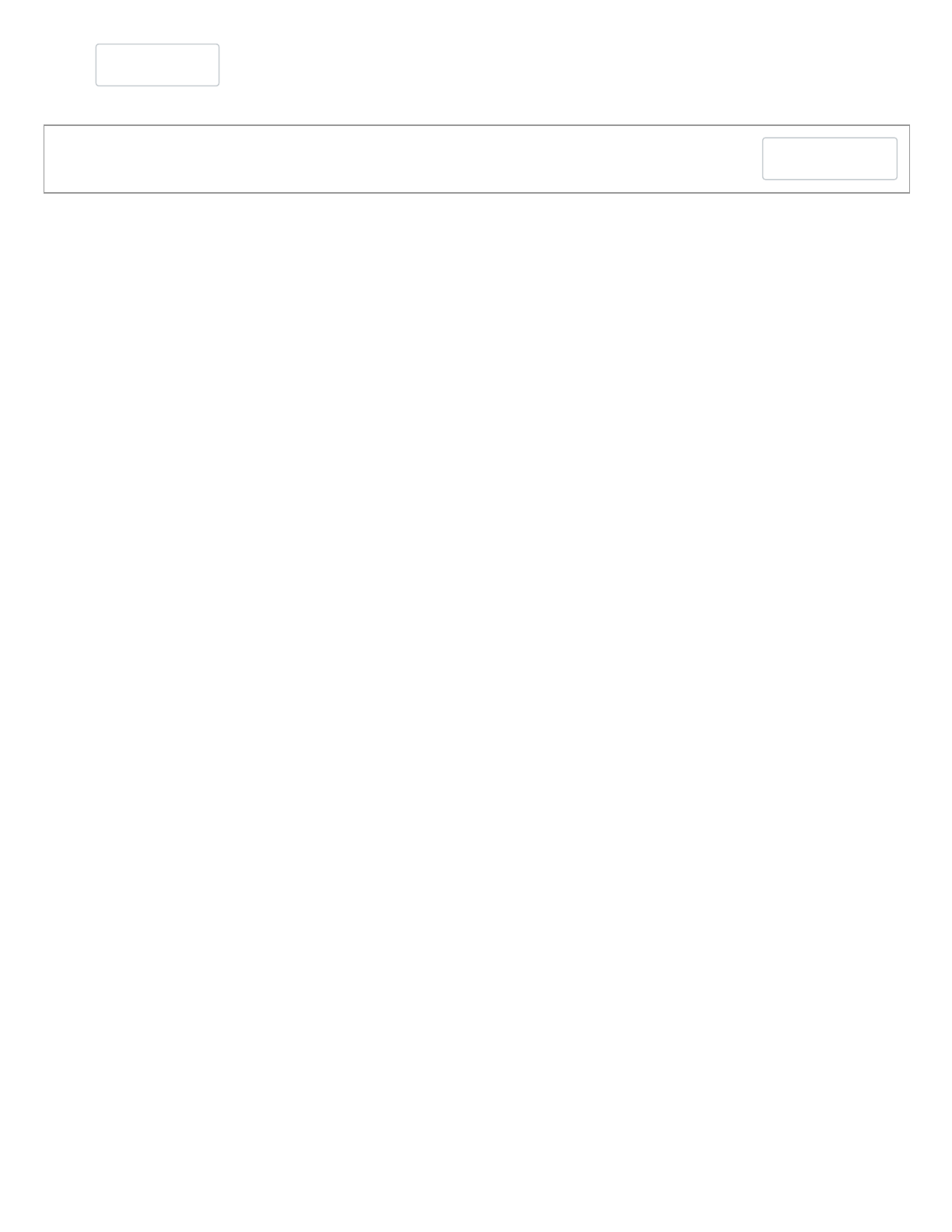
2/26/2019 Quiz: Lab 06
https://cilearn.csuci.edu/courses/6978/quizzes/15478/take/questions/239479 4/4
Notsaved
Previous
SubmitQuiz