1 Investigating CPU Instructions
User Manual: Pdf
Open the PDF directly: View PDF .
Page Count: 4
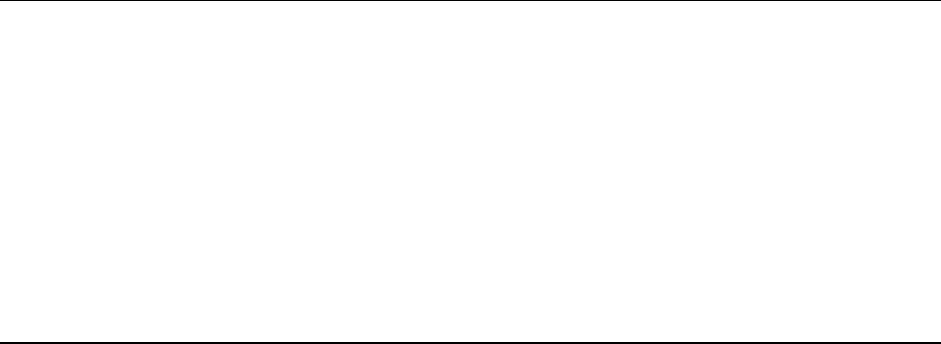
InvestigatingCPUInstructions
Objectivesofthistutorial
Attheendofthislabyoushouldbeableto:
EnterCPUinstructionsusingtheCPUsimulator
DescribetheeffectofcompareinstructiononCPUstatusflagsZandN
ConstructaloopandexplainjumpinstructionsthatuseZandNflags
Usedirectaddressingtoaccessmemorylocation(s)
Useindirectdirectaddressingtoaccessmemorylocation(s)
Constructasubroutineandcallit
Passparameter(s)tothesubroutine
Specialinstructions
Saveyourworkatregularintervals.
TheCPUsimulatorisworkinprogresssoitmaycrashfromtimetotime.Ifthishappens
thenrestartitandloadyourlatestcode,hencetheimportanceoftheabovestatement!
Askifyouneedhelpwithanyaspectofthistutorial.
Thesimulatoristheretohelpyouunderstandthetheorycoveredinlecturessomake
themostofit.
1
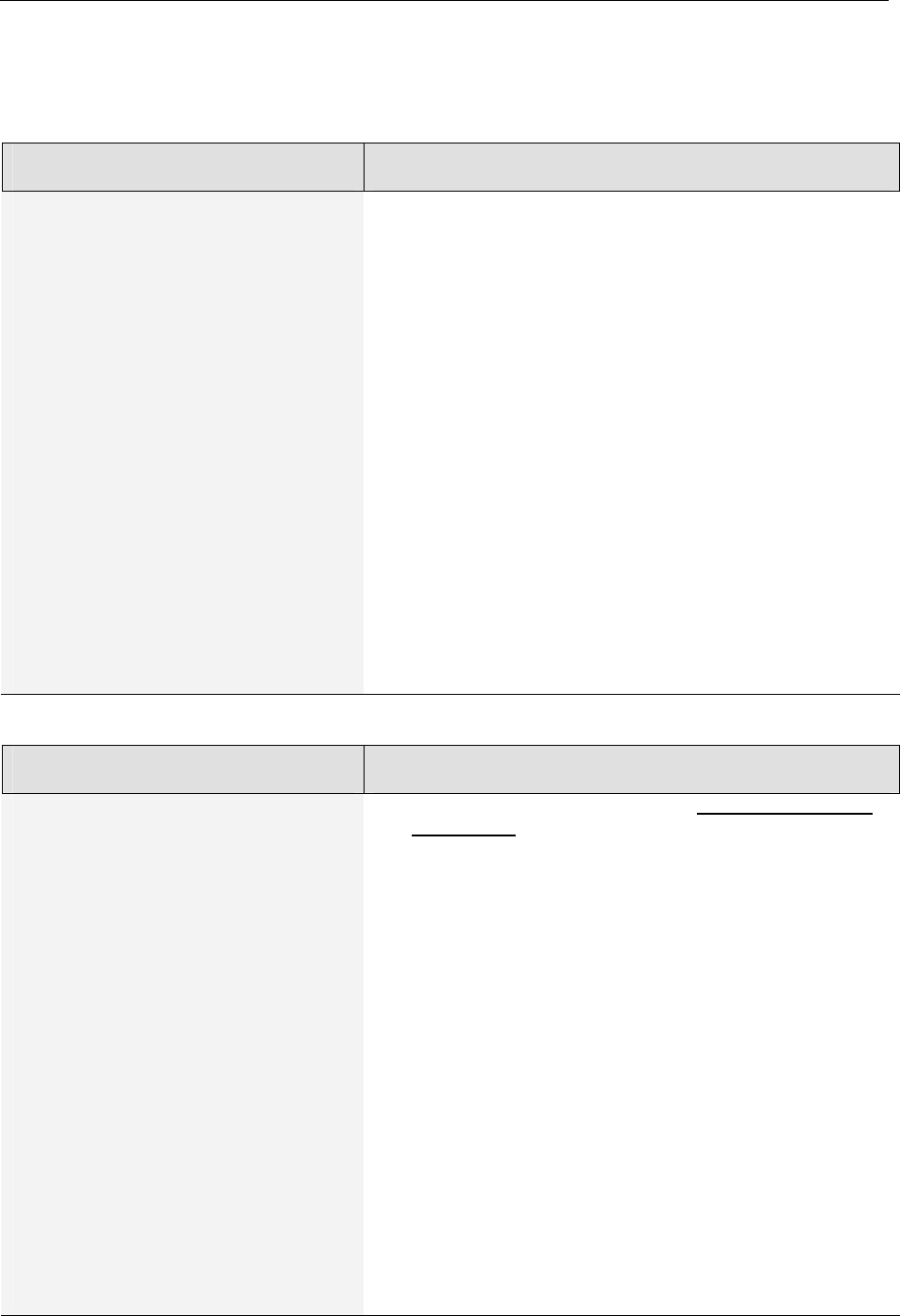
Exercises
Learningobjectives:ToenterCPUinstructionsanddescribeeffectofcompareinstructionon
CPUstatusflagsZandN.
IntheoryInpractice
TheCPUinstructionsetsareoften
groupedtogetherintocategories
containinginstructionswithrelated
functions.
Thisexercisefamiliarisesyouwith
thewaytheinstructionsareentered
usingthesimulator.
Themostencounteredinstructions
involvedatamovements,e.g.the
MOVinstruction.
Anothercommoninstructionisthe
comparisoninstruction,e.g.CMP.
Thisinstructionsetsorresetsthe
statusflagsZandNasarecordof
theresultofthecomparison
operation.
1. Enter the following code and run it:
MOV #1, R01
MOV #2, R02
ADD R01, R02
2. Add the following code and note the states of the status
flags Z and N after each compare instruction is executed:
CMP #3, R02 Z N
CMP #1, R02 Z N
CMP #4, R02 Z N
Explainyourobservationsofthestatesofthestatus
flagsafterthecompareinstructionsabove:
** Now save the above code! **
Learningobjectives:ToconstructaloopandexplainjumpinstructionsthatuseZandNflags
IntheoryInpractice
Thecomputerprogramsoften
containloopswherethesame
sequenceofcodeisrepeatedly
executeduntilorwhilecertain
conditionismet.Loops(oriterative
statements)arethemostuseful
featuresofprogramminglanguages.
Attheinstructionlevel,loopsuse
conditionaljumpinstructionsto
jumpbacktothestartoftheloopor
tojumpoutoftheloop.
Thisexerciseiscreatedto
demonstratetheuseofthejump
instructionwhichoftenusesthe
resultofacompareinstruction.Such
jumpinstructionsusetheZandthe
Nstatusflagstojumpornotjump.
3. Add the following code and run it (ask your tutor how to
enter a label):
MOV #0, R01
Label1
ADD #1, R01
CMP #3, R01
JLT $Label1
Summarize what the above code is doing in plain
English:
4. Modify the above code such that R01 is incremented by 1
until it reaches the value of 4. Copy the code below:
** Now save the above code! **
2

Learningobjectives:Tousedirectaddressingtoaccessmemorylocation(s)
IntheoryInpractice
Althoughinstructionsmovingdata
toorfromregistersarethefastest
instructions,itisstillnecessaryto
movedatainoroutofthemain
memory(RAM)whichisamuch
slowerprocess.
Examplesofinstructionsusedto
storeintoorgetdataoutofmemory
areexploredhere.Themethodused
hereusesthedirectaddressing
method,i.e.thememoryaddressis
directlyspecifiedintheinstruction
itself.
5. Add the following code and run:
STB #h41, 16
LDB 16, R03
ADD #1, R03
STB R03, 17
Make a note of what you see in the program’s data area:
What is the significance of h in h41 above?
Modify the above code to store the next two characters in
the alphabet. Write down the modified code below:
** Now save the above code! **
Learningobjective:Touseindirectaddressingtoaccessmemorylocation(s)
IntheoryInpractice
Therearecircumstanceswhichmake
directaddressingunsuitableand
inflexiblemethodtouse.
Inthesecasesindirectaddressingis
amoresuitableandflexiblemethod
touse.Inindirectaddressing,the
addressofthememorylocationis
notdirectlyincludedinthe
instructionbutisstoredinaregister
whichistheusedintheinstruction.
Thisexerciseintroducesanindirect
methodofaccessingmemory.Thisis
calledregisterindirectaddressing.
Thereisalsothememoryindirect
addressingbutthisisleftasan
exerciseforyou.
6. Add the following code and run:
Label2
MOV #16, R03
MOV #h41, R04
Label3
STB R04, @R03
ADD #1, R03
ADD #1, R04
CMP #h4F, R04
JNE $Label3
Make a note of what you see in the program’s data area:
Explain the significance of @ in @R03 above:
** Now save the above code! **
3

Learningobjective:Toconstructasubroutineandcallit
IntheoryInpractice
Anotherveryusefulfeatureof
programminglanguagesis
subroutines.Thesecontain
sequencesofinstructionswhichcan
beexecutedmanytimes.Ifa
subroutinewasnotavailablethe
samesequenceofinstructions
wouldhavebeenrepeatedmany
timesincreasingthecodesize.
Atinstructionlevel,asubroutineis
calledbyaninstructionsuchasCAL.
Thiseffectivelyisajumpinstruction
butitalsosavestheaddressofthe
nextinstruction.Thisislaterusedby
asubroutinereturninstruction,e.g.
RETtoreturntotheprevious
sequenceofinstructions.
7. Add the following code but do NOT run it yet:
MSF
CAL $Label2
Now convert the code in (6) above into a subroutine by
inserting a RET as the last instruction in the subroutine.
Make a note of the contents of the PROGRAM STACK
after the instruction MSF is executed (see tutor what to
do to facilitate this observation):
Make a note of the contents of the PROGRAM STACK
after the instruction CAL is executed:
What is the significance of the additional information?
** Now save the above code! **
Learningobjective:Topassparameter(s)tothesubroutine
IntheoryInpractice
Usefulastheyarethe
subroutinesarenotvery
flexiblewithouttheuseofthe
subroutineparameters.These
arevaluespassedtothe
subroutinetobeconsumed
insidethesubroutine.
Thecommonmethodof
passingparameterstothe
subroutinesisusingthe
programstack.The
parametersarepushedonthe
stackbeforethecall
instruction.Thesearethen
poppedoffthestackinsidethe
subroutineandconsumed.
Thisexerciseiscreatedto
demonstratethismechanism.
8. Let’s make the above subroutine a little more flexible. Suppose
we wish to change the number of characters stored when
calling the subroutine. Modify the calling code in (7) as below:
MSF
PSH #h60
CAL $Label2
Now modify the subroutine code in (6) as below and run the
above calling code:
Label2
MOV #16, R03
MOV #h41, R04
POP R05 Å
Label3
STB R04, @R03
ADD #1, R03
ADD #1, R04
CMP R05, R04 Å
JNE $Label3
RET
Add a second parameter to change the starting address of the
data as a challenge and write the code in the box above!
** Now save the above code! **
4