Lab 2 Instructionsx Instructions
User Manual: Pdf
Open the PDF directly: View PDF .
Page Count: 3
Real‐TimeProgramming,Lab2Instructions March2017,ÖrebroUniversity
pg.1
Lab 2: Breathing LED, Buttons
Get familiar with and implement PWM, practice with buttons
FarhangNemati,March2017
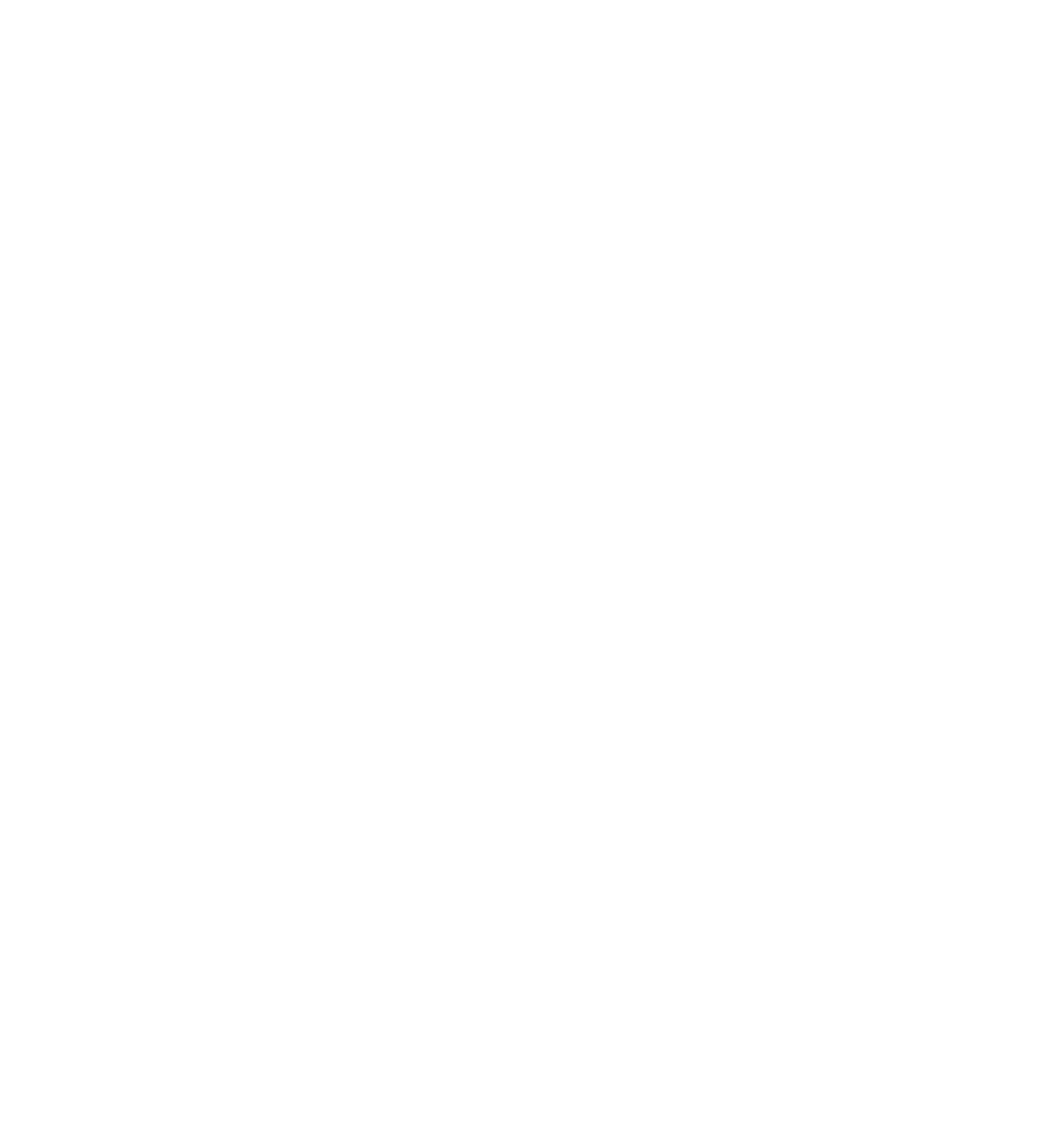
Real‐TimeProgramming,Lab2Instructions March2017,ÖrebroUniversity
pg.2
InthislabyouwilllearnandpracticetheconceptofPulseWidthModulation(PWM).Thenyouwilluse
ittoimplementabreathingLED.YouwilllateronneedPWMinLab4aswell.Youwillalsolearnhowtowork
withbuttons.
A. Breathing LED
A.1.
ReusetheblinkingLEDprogramyouimplementedinLab1.Insteadofsleep()useclock_nanosleep()
functiontohavemoreprecisedelays.ChangetheprogramsothattheLEDisturnedonfor20ms(milliseconds)
anditisoffforanother20ms.Lettheprogramdothecycleforenoughnumberoftimes(e.g.,2000times).Now
changetheprogramsothattheLEDisturnedonfor10msandisturnedofffor20ms.Finallychangetheprogram
sothattheLEDisonfor5msandisofffor20ms.Whatisthedifferenceamongthealternativeswithregardto
theshiningstrength(brightness)oftheLED?Why?
PulseWidthModulation
The mainuseofPWMistocontrolthepowersuppliedtoelectricaldevices,especiallytoinertialloads
suchasmotorsetc.Assumeamotorisgoingtobecontrolledbyanoutputthatsuppliespowertothemotor.
Whentheoutputishighthemotorwillrunwithaconstantspeed.Whatwouldyoudosothatthemotorruns
withhalfofthisspeed?Iftheoutputishighforadurationoftime,sayt1andislowfort1timeunitsthemotor
willrunfort1timeunitsanditwillstopforanothert1timeunits.Ifthisprocessiscontinuedthemotorwillbe
runningfor50%oftime,thusitwillhave50%offullspeed.
Forexampleifthepowerisonfor1secondandisofffor1secondthemotorwillruneveryothersecond
anditsaveragespeedwouldbe50%ofitsfullspeed.However,thedurationof1secondistoolongandonecan
clearlyseethatrunningandstoppingofthemotoristoodiscrete.Ifthefrequencyofrunningandstoppingthe
motor(turningonandoffthepoweroutput)isdecreasedenoughonewillnotnoticethatrunningandstopping
isdiscretebutitwillseemmorecontinues.Forexampleifthepoweroutputisonfor10msandisofffor10ms
themotorwillseemtoruncontinuously.Itwillstillrunwith50%ofitsfullspeedbecauseitrunsforonly50%
oftime.Itseemscontinuesbecauseweincreasedthefrequencyofturningonandoff.Whatwedoistohave
pulsesof20msandduringitthemotorisonfor10msandofffor10ms,i.e.,for50%ofthepulsethemotoris
onanditisoffforanother50%ofeachpulse.Whatwouldyoudotorunthemotorwith25%ofitsfullspeed?
APWMhasafrequencywhichindicatesthelengthofthepulse.ForexampleifthefrequencyofPWMis
100HZ(100pulsespersecond)itmeansthelengthofeachpulseis1/100second=10ms.Thedurationoftime
duringwhichtheoutputishigh(forexample25%)iscalleddutypercent.Forthemotorexamplementioned
above,tocontrolthespeedofamotorwecanhaveaPWMthathasapulselengthof20ms(thefrequencyis
50HZ).Ifwewantthemotortorunwith25%offullspeedwehavetosetthedutypercentto25%.Thismeans
duringofeachpulse(20ms)theoutputisonfor25%ofthepulse(for5ms)andisofffor75%ofthepulse(15ms).
A.2.
ImplementPWM.Downloadthecode(pwm.handpwm.c)fromBlackboard.Implementfunctions
pwmCreate()andpwmPulse()inpwm.c.pwmCreate()isusedtocreateaPWMonaGPIOpinwithafrequencyand
pwmPulse()isusedtoissueadutypercentonthepin.WhenyoucreateaPWMonaGPIOwithfrequency,sayf,
thepinwillbesettoanoutputpinandwhenpwmPulse()iscalledforagivendutypercent,sayd,theGPIOpin
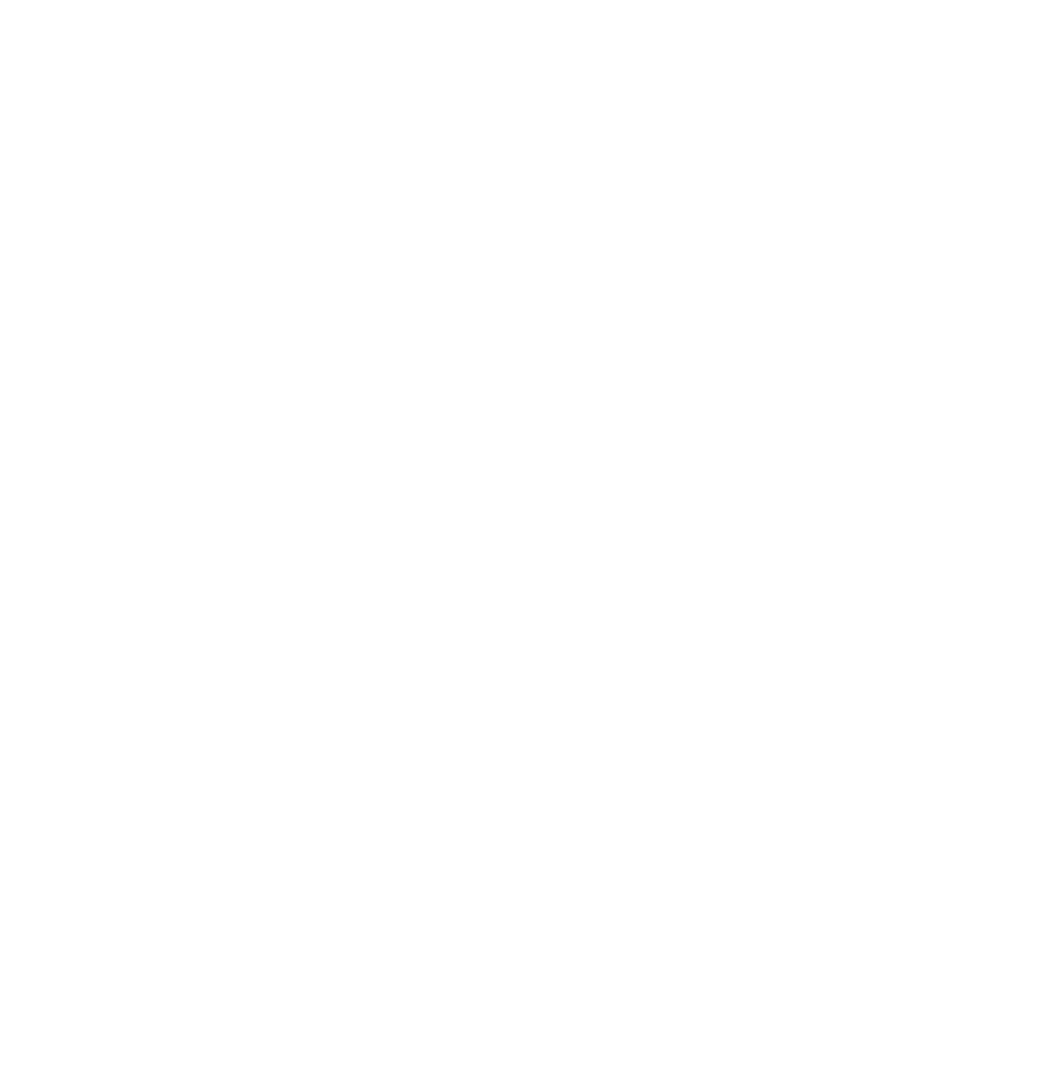
Real‐TimeProgramming,Lab2Instructions March2017,ÖrebroUniversity
pg.3
shouldbehighford%ofthelengthofapulsethathasfrequencyfanditshouldbelowfor(100‐d)%ofthe
lengthofthepulse.Thelengthofapulsewithfrequencyoffis1/fsecond.CallpwmDestroy()onanyPWMyou
createafteryouarefinishedwithit
A.3.
UsethePWMforimplementingabreathingLED.ThebrightnessofaLEDhastograduallyincreasefrom
0%(off)to100%(fullyshining)andthenthebrightnesshastograduallydecreasefrom100%backto0%.Repeat
thisinaloop.ChooseagoodenoughfrequencyforPWMsothatit’scontinuousenoughthatitlookslikethe
LEDisbreathing.
B. Using Buttons
Thereare2buttons(atleast)alreadyinstalledonyourbreadboard.ReadSection“UsingaGPIOpinfor
buttonsandswitches”inthedocumentforGeneralInstructions(itisonBlackboard).Usepull‐upbuttonshere.
Rememberthatthevalueofaninputpinthatapull‐upbuttonisconnectedtobydefaultishigh.Whenthe
buttonispushedthevalueoftheinputpinbecomeslow.Onepinofeachbuttonisalreadyconnectedtothe
GND.TheotherpinhastobeconnectedtoaGPIOpinwhichhastobecreatedasapull‐upinputpin.
B.1.
ImplementaprogramwhereeachoftheLEDsarecontrolledbyoneofthebuttons.Whenabuttonis
pusheditscorrespondingLEDhastobeturnedon.Whenthebuttonispushedagain,theLEDshouldturnoff.
B.2.
InthisSectionyouwillreusethePWMyouimplementedinSectionA.Implementaprogramwherethe
brightnessofaLEDsiscontrolledbyabutton.EachLEDhastohave5states;state1wheretheLEDisturned
off,state2wheretheLEDshineswith25%ofitsfullbrightness,state3wheretheLEDshines50%,state4where
theLEDshines75%,andstate5wheretheLEDshinesfully(100%).BydefaulttheLEDhastobeoff.Whenits
correspondingbuttonispushed,theLEDshouldgotostate1,andwhenthebuttonispushedagainitwillgoto
state2,andtostate3whenthebuttonispushedforthethirdtime,tostate4whenthebuttonispushedfor
4thtime,tostate5whenitispushedfor5thtime,andfinallyitshouldgobacktostate1(turnedoff)whenthe
buttonispushedforthe6thtime.It’senoughifyouimplementthisonlyforoneLEDandonecorresponding
button.
Examination
Topassthelabhandinashortreportandyourcode(onlyinBlackboard!).Youalsoneedto
demonstratetheprogramsforthelabassistantinoneofthelabsessions.Thelabassistantmayaskyou
questionsrelatedtolabtask.