Lab2 Instructions
User Manual: Pdf
Open the PDF directly: View PDF .
Page Count: 3
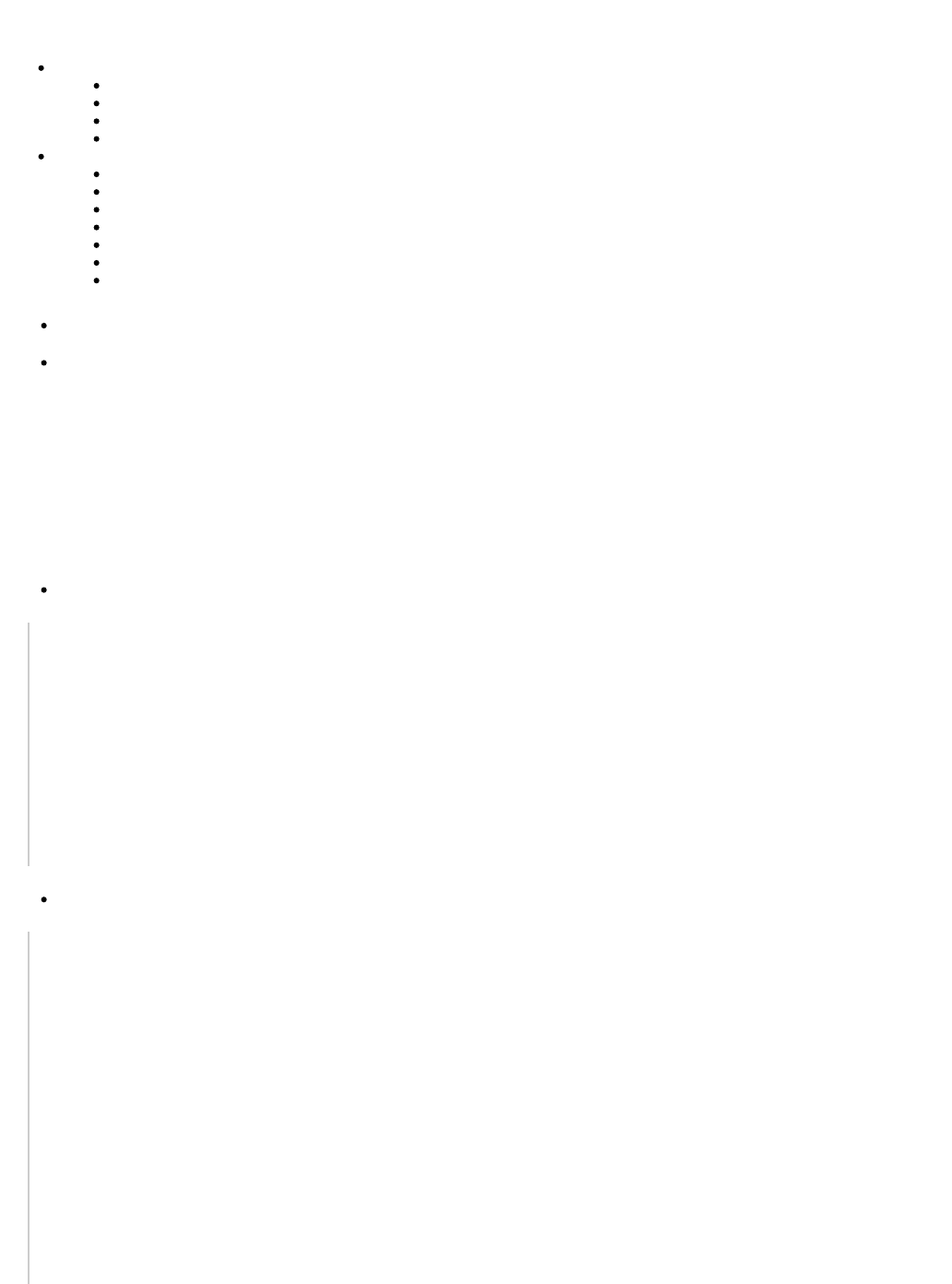
5. Assignment 2 - XML, REST JAX-RS
Customer object with attributes firstname, lastname, email
XML Data sample
Create com.rest.domain.Customer in eclipse project
Create Resourse com.rest.resource.CustomerResource
Show CRUD using CURL with XML data
Assignment 2 - Optional
Prereqs
Create domain object - Podcast
Create XML Data Sample
Create CURL commands for POST, GET, PUT and DELETE with XML data
Create PodcastResource for POST, GET, PUT and DELETE
Run it in Eclipse using JETTY or Tomcat
Execute CURL commands and verify results in POST, GET, PUT, GET, DELETE and GET order.
Customer object with attributes firstname, lastname, email
XML Data sample
<customer>
<firstname>Bill</firstname>
<lastname>Burke</lastname>
<email>bill.burke@gmail.com</email>
</customer>
Create com.rest.domain.Customer in eclipse project
package com.rest.domain;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlElement;
@XmlRootElement(name="customer")
public class Customer {
// Maps a object property to a XML element derived from property name.
@XmlElement public int id;
@XmlElement public String firstname;
@XmlElement public String lastname;
@XmlElement public String email;
}
Create Resourse com.rest.resource.CustomerResource
package com.rest.resource;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.atomic.AtomicInteger;
import .rs.Consumes;javax.ws
import .rs.DELETE;javax.ws
import .rs.GET;javax.ws
import .rs.POST;javax.ws
import .rs.PUT;javax.ws
import .rs.Path;javax.ws
import .rs.PathParam;javax.ws
import .rs.Produces;javax.ws
import .rs.WebApplicationException;javax.ws
import .rs.core.Response;javax.ws
@Path("customers")
public class CustomerResource {
// ConcurrentHashMap - A hash table supporting full concurrency of retrievals and adjustable expected concurrency for
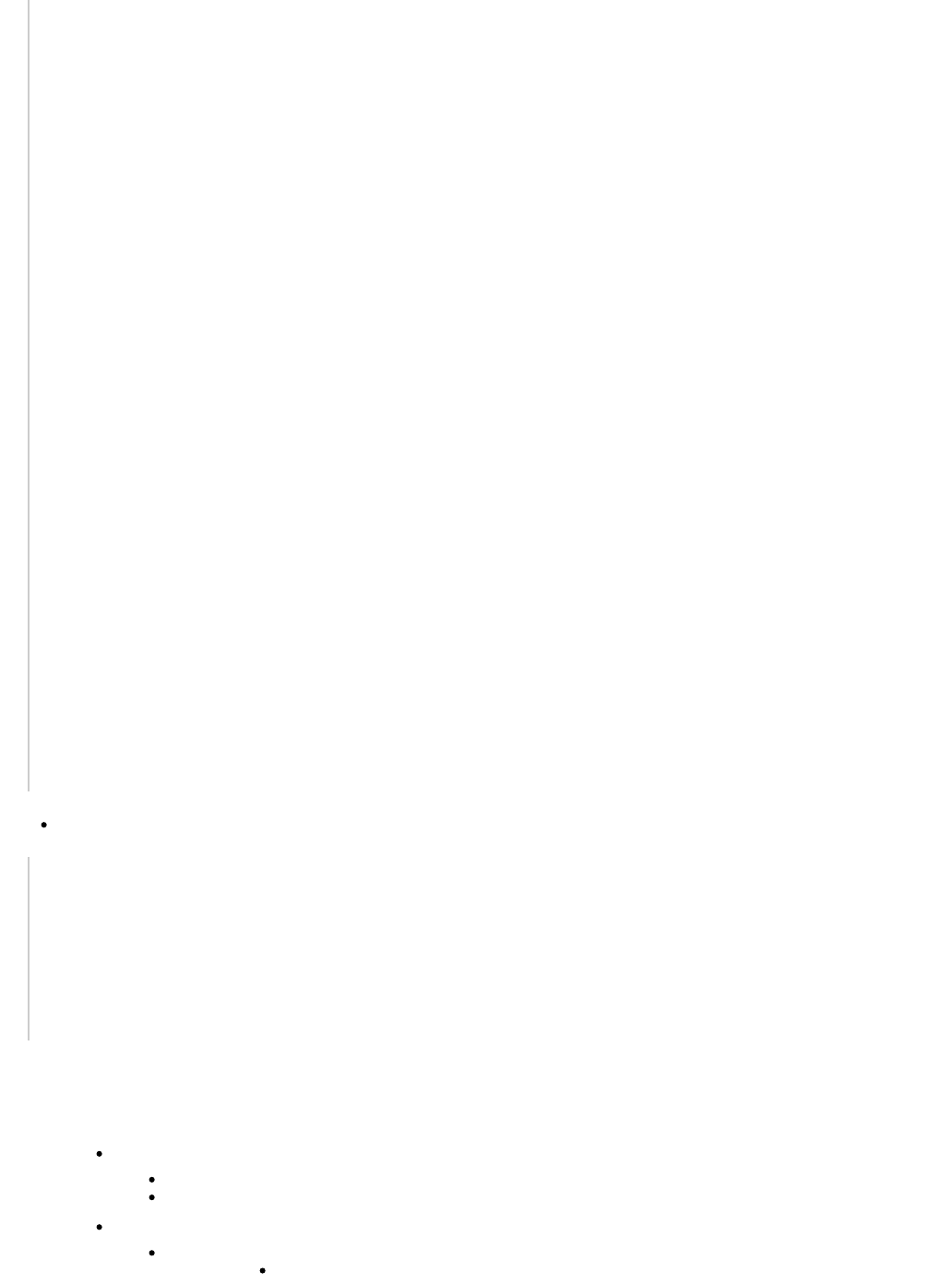
updates.
static private Map<Integer, Customer> customerDB = new ConcurrentHashMap<Integer, Customer>();
// An AtomicInteger is used in applications such as atomically incremented counters
private AtomicInteger idCounter = new AtomicInteger();
@POST
@Consumes("application/xml")
public Customer createCustomer(Customer customer) {
= idCounter.incrementAndGet();customer.id
customerDB.put( , customer);customer.id
return customer;
}
@GET
@Path("{id}")
@Produces("application/xml")
public Customer getCustomer(@PathParam("id") int id) {
Customer customer = customerDB.get(id);
return customer;
}
@PUT
@Path("{id}")
@Consumes("application/xml")
public void updateCustomer(@PathParam("id") int id, Customer update) {
Customer current = customerDB.get(id);
current.firstname = update.firstname;
current.lastname = update.lastname;
current.email = update.email;
customerDB.put( , current);current.id
}
@DELETE
@Path("{id}")
public void deleteCustomer(@PathParam("id") int id) {
Customer current = customerDB.remove(id);
if (current == null) throw new WebApplicationException(Response.Status.NOT_FOUND);
}
}
Show CRUD using CURL with XML data
curl -H "Content-Type: application/xml" -X POST -d
"<customer><firstname>Bill</firstname><lastname>Burke</lastname><email>bill.burke@ </email></customer>" gmail.com http:/
/localhost:8080/lab2/rest/customers
curl -H "Content-Type: application/xml" -X GET http://localhost:8080/lab2/rest/customers/1
curl -H "Content-Type: application/xml" -X PUT -d
"<customer><firstname>Ed</firstname><lastname>Burke</lastname><email>ed.burke@ </email></customer>" gmail.com http://l
ocalhost:8080/lab2/rest/customers/1
curl -H "Content-Type: application/xml" -X DELETE http://localhost:8080/lab2/rest/customers/1
Assignment 2 - Optional
Prereqs
Lab1 is setup correctly
CURL is installed
Create domain object - Podcast
A podcast will have the following attributes or properties :
feed – url feed of the podcast :String
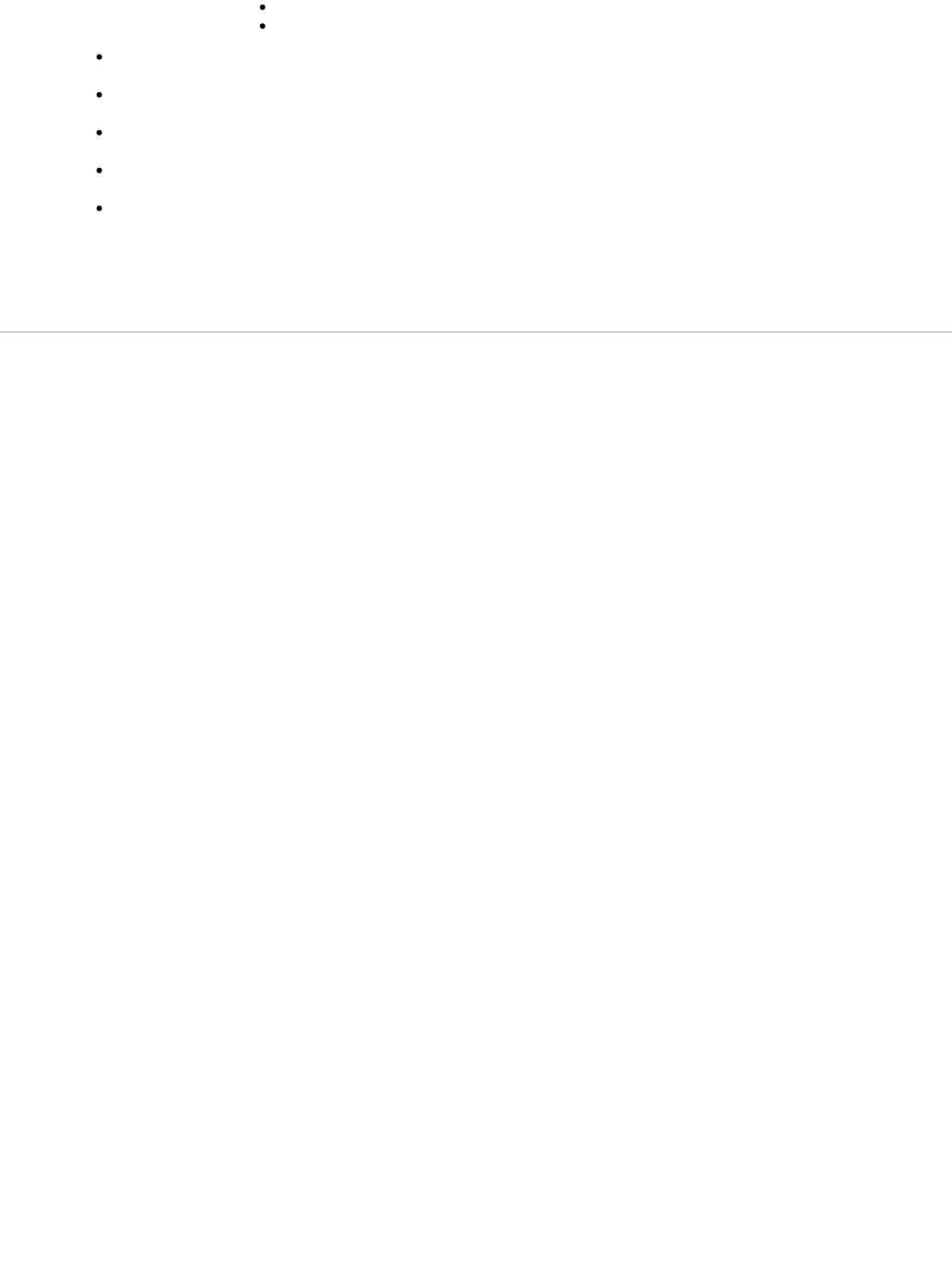
title – title of the podcast : String
description – a short description of the podcast : String
Create XML Data Sample
Create CURL commands for POST, GET, PUT and DELETE with XML data
Create PodcastResource for POST, GET, PUT and DELETE
Run it in Eclipse using JETTY or Tomcat
Execute CURL commands and verify results in POST, GET, PUT, GET, DELETE and GET
order.
© 2016 Sanjay Patni